Hello visitor, Welcome to our new blog on Flutter. In this post, we will code a QR Generator Android Application in 100 lines of dart code. So, as we already know get your laptops ready with a new flutter project created and opened in your favorite IDE (Mine’s VS Code❤).
#100LinesOfCode
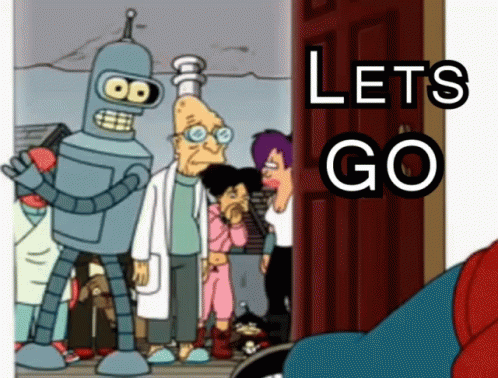
- Let’s start doing this, open main.dart in your editor.
- We will use an Open API to generate QR code, which takes data as input and returns us the QR Code in .png format.
- So, we need to make an HTTP request to get our QR code from generator service.
- We can use Image.network() constructor of Image Widget in Flutter, which makes a http call, and renders the image bytes of png file on our screen.
- We are all set, let’s just have a look on the final output before we code it.

- Now, let’s code this in #100Lines. Open your main.dart and replace with the following Code.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
bool isPressed = false;
var data;
@override
Widget build(BuildContext context) {
TextEditingController _controller = TextEditingController();
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.purple[300],
title: Text("QR Code Generator"),
),
floatingActionButton: RaisedButton(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(15))),
color: Colors.purple[300],
child: Text("Generate QR"),
onPressed: () {
setState(() {
data = _controller.text;
isPressed = true;
});
},
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat,
body: Center(
child: Column(children: <Widget>[
Container(
padding: EdgeInsets.fromLTRB(20, 20, 20, 100),
child: TextField(
controller: _controller,
decoration: InputDecoration(
focusedBorder: OutlineInputBorder(
borderSide: BorderSide(color: Colors.purple[300]),
borderRadius: BorderRadius.all(Radius.circular(15)),
),
border: OutlineInputBorder(
borderRadius: BorderRadius.all(Radius.circular(15)),
),
hintText: "Enter the Data to generate QR code (eg. url)"),
)),
isPressed
? Image.network(
"https://api.qrserver.com/v1/create-qr-code/?size=150x150&data=$data",
)
: SizedBox(),
SizedBox(
height: 20,
),
isPressed
? Column(
children: [
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Icon(
Icons.check,
color: Colors.green,
size: 30,
),
Text(
"QR Generated..!",
style: TextStyle(fontSize: 20),
),
]),
Text(
"Take a ScreenShot and Share it..😜",
style: TextStyle(fontSize: 20),
)
],
)
: SizedBox()
])),
);
}
}
Widget Tree

The above Widget tree, gives us an overview of how we have code our screen in Flutter.
Want to see one such view for your own flutter application, open the Flutter extension in your VS Code in the left pane and have a look on yours.

Let’s check if it worked. Here’s the QR Code of our blogs home page. Scan it in your phone and let us know whether it worked in the comments.

Follow our Facebook page at HelloWorldFB. To join our team and Help World Learn, have a look at our team page and register yourself.
You can also download the main.dart file from our GitHub organization.
Let’s meet again on our learning journey. Until then stay safe, stay home. Cheers..✌✌